Production vs development mode: coding nightmare
Originally published: 7 January 2024
Everything worked 100% on dev mode, but production was failing
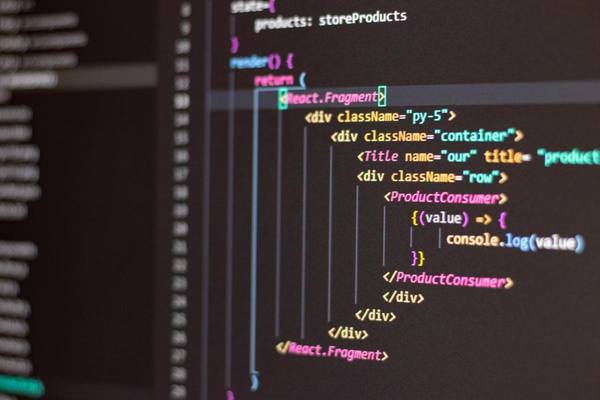
This code worked 100% in dev mode, but failed horribly in production mode
---
// Import necessary utilities
import { getCollection } from 'astro:content';
import MarkdownPostLayout from '../../layouts/MarkdownPostLayout.astro';
import SharePage from '../../components/SharePage.astro';
export async function getStaticPaths() {
const blogEntries = await getCollection('posts');
return blogEntries.map(entry => ({
params: { slug: entry.slug }, props: { entry },
}));
}
const { entry } = Astro.props;
const { Content } = await entry.render();
// Fetching posts and sorting them
const allPosts = (await getCollection('posts'))
.sort((a, b) => new Date(b.data.pubDate).getTime() - new Date(a.data.pubDate).getTime());
const fullSlug = Astro.url.pathname;
console.log(fullSlug)
const currentSlug = fullSlug.split('/').pop() || '';
// console.log("Current Slug:", currentSlug);
// allPosts.forEach(post => console.log("Post Slug:", post.slug));
const findCurrentPostIndex = (slug: string) => {
return allPosts.findIndex(post => post.slug === slug);
};
const currentIndex = findCurrentPostIndex(currentSlug);
console.log("Current Index:", currentIndex);
const previousPost = allPosts[currentIndex - 1];
const nextPost = allPosts[currentIndex + 1];
---
<MarkdownPostLayout frontmatter={entry.data}>
<Content />
<div class="w-full mx-auto text-center inline-flex no-underline mb-5">
{previousPost && (
<a href={`/posts/${previousPost.slug}`} class="pt-2 bg-gray-100 hover:bg-gray-800 hover:text-white rounded-lg no-underline w-1/2 md:w-3/4 lg:w-1/2 inline-block text-black mr-4">
<p class="no-underline mx-auto font-semibold">⬅️ Previous Post </p>
<p class="px-1">{previousPost.data.title}</p>
</a>
)}
{nextPost && (
<a href={`/posts/${nextPost.slug}`} class="pt-2 bg-gray-100 hover:bg-gray-800 hover:text-white rounded-lg no-underline w-1/2 md:w-3/4 lg:w-1/2 inline-block text-black ml-4">
<p class="no-underline mx-auto font-semibold">Next Post ➡️</p>
<p class="px-1">{nextPost.data.title}</p>
</a>
)}
</div>
<SharePage />
<script type="module">
import { updateReadCount } from '/firebaseConfig.js';
document.addEventListener('DOMContentLoaded', async () => {
const slug = '${currentSlug}';
const readCount = await updateReadCount(slug);
document.getElementById('read-count').textContent = `Read Count: ${readCount}`;
});
</script>
<div id="read-count">Read Count: Loading...</div>
</MarkdownPostLayout>
Turned out it was the ‘full slug’ that was the issue
changing to
---
// Import necessary utilities
import { getCollection } from 'astro:content';
import MarkdownPostLayout from '../../layouts/MarkdownPostLayout.astro';
import SharePage from '../../components/SharePage.astro';
export async function getStaticPaths() {
const blogEntries = await getCollection('posts');
return blogEntries.map(entry => ({
params: { slug: entry.slug }, props: { entry },
}));
}
const { entry } = Astro.props;
const { Content } = await entry.render();
// Fetching posts and sorting them
const allPosts = (await getCollection('posts'))
.sort((a, b) => new Date(b.data.pubDate).getTime() - new Date(a.data.pubDate).getTime());
// Use params for getting the current slug
const currentSlug = Astro.params.slug;
console.log("Current Slug:", currentSlug);
const findCurrentPostIndex = (slug: string) => {
return allPosts.findIndex(post => post.slug === slug);
};
const currentIndex = findCurrentPostIndex(currentSlug);
console.log("Current Index:", currentIndex);
const previousPost = allPosts[currentIndex - 1];
const nextPost = allPosts[currentIndex + 1];
---
<MarkdownPostLayout frontmatter={entry.data}>
<Content />
<div class="w-full mx-auto text-center inline-flex no-underline mb-5">
{previousPost && (
<a href={`/posts/${previousPost.slug}`} class="pt-2 bg-gray-100 hover:bg-gray-800 hover:text-white rounded-lg no-underline w-1/2 md:w-3/4 lg:w-1/2 inline-block text-black mr-4">
<p class="no-underline mx-auto font-semibold">⬅️ Previous Post </p>
<p class="px-1">{previousPost.data.title}</p>
</a>
)}
{nextPost && (
<a href={`/posts/${nextPost.slug}`} class="pt-2 bg-gray-100 hover:bg-gray-800 hover:text-white rounded-lg no-underline w-1/2 md:w-3/4 lg:w-1/2 inline-block text-black ml-4">
<p class="no-underline mx-auto font-semibold">Next Post ➡️</p>
<p class="px-1">{nextPost.data.title}</p>
</a>
)}
</div>
<SharePage />
<script type="module">
import { updateReadCount } from '/firebaseConfig.js';
document.addEventListener('DOMContentLoaded', async () => {
const slug = '${currentSlug}';
const readCount = await updateReadCount(slug);
document.getElementById('read-count').textContent = `Read Count: ${readCount}`;
});
</script>
<div id="read-count">Read Count: Loading...</div>
</MarkdownPostLayout>
worked perfectly and now I have my in page (prev/next post) navigation back!
Read Count: 0