Is there an alternative to Astro/Image?
Originally published: 7 January 2024
Last updated: 8 January 2024
Prefetching images from Astro/Image is all but impossible. is there another way?
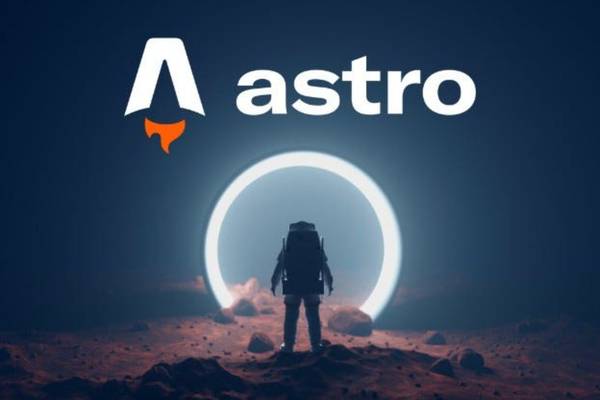
Update: I found a way to prefetch Dynamic images using Astro/Image
and Astro/Picture
! Check out the new guide here.
Are you using Astro/Image for image optimization in your web development projects and wondering if there might be a more flexible alternative? While Astro/Image is a popular choice for optimizing images in Astro applications, it’s worth exploring whether there are other approaches that provide more control and customization options, especially when it comes to prefetching images.
The Challenge with Prefetching Images in Astro/Image
One common concern when using Astro/Image is prefetching images. Prefetching is a technique that allows browsers to fetch resources such as images in advance, which can help improve page loading times. However, prefetching images from Astro/Image can be challenging due to some limitations:
Preload Is Retired
The preload
attribute, which was commonly used for prefetching resources, has been retired and replaced with the “speculation rules” in modern browsers like Chrome. This change means that prefetching resources now relies on new mechanisms, and the behavior can vary between browsers.
For more information, you can refer to the official documentation.
Default Prefetch Behavior
By default, prefetching in Astro only pulls the HTML, not the assets like images or CSS files. To prefetch images, you need to have the URL of the image and prefetch it separately.
This process can become even trickier when dealing with dynamic imports or complex web applications.
Alternatives to Astro/Image
If you’re looking for alternatives to Astro/Image, it’s essential to understand the trade-offs and choose the one that best suits your project’s needs:
1. Next/Image
Next/Image is another popular choice for image optimization, but it differs from Astro/Image in a significant way. Instead of generating optimized images at build time, Next/Image processes images on the client side. While this can be convenient, it may consume more bandwidth, especially if your site has many images. Additionally, it’s important to note that Cloudflare does not support Next/Image.
To illustrate, here’s an example of how you can use Next/Image for image optimization:
import Image from 'next/image'
function MyComponent() {
return (
<div>
<Image
src="/cute-bunnies.jpg"
alt="Cute Bunnies"
width={300}
height={200}
/>
</div>
)
}
2. DIY Image Optimization
Consider implementing your custom image optimization script using tools like Sharp. This approach allows you to have full control over image optimization, including size, format, and quality.
Here’s an example script to get you started:
// Import necessary libraries
import sharp from 'sharp'
import fs from 'fs'
import path from 'path'
// Define sizes and formats
const sizes = [200, 300, 450, 600, 750, 900, 1200]
const formats = ['avif', 'webp']
const sourceDirectory = 'src/images/'
const outputDirectory = 'public/images/'
// Iterate through image files
fs.readdir(sourceDirectory, (err, files) => {
if (err) {
console.error("Error reading directory:", err);
return;
}
files.forEach(file => {
const sourceImagePath = path.join(sourceDirectory, file);
if (fs.statSync(sourceImagePath).isFile()) {
sizes.forEach(size => {
formats.forEach(format => {
const outputFilePath = path.join(outputDirectory, `${path.parse(file).name}-${size}.${format}`);
sharp(sourceImagePath)
.resize(size)
.toFormat(format)
.toFile(outputFilePath)
.then(() => {
console.log(`Generated: ${outputFilePath}`);
})
.catch(err => {
console.error(`Error processing ${outputFilePath}: ${err}`);
});
});
});
}
});
});
While this approach gives you control, it may generate a large number of images for each source image, which could be overwhelming for your images folder. You can adjust the sizes to a more manageable set as needed.
The Verdict: Is It Worth the Effort?
The big question is whether the effort of implementing a custom image optimization solution is worth it. While it provides control and customization, the actual benefit in terms of user experience and performance may not be significant.
Prefetching images from Astro/Image may save some loading time, but the actual time saved depends on various factors, as mentioned earlier. In practice, prefetching a 50KB image might save anywhere from a few milliseconds to a few tenths of a second. Therefore, it’s essential to weigh the trade-offs and consider whether the hassle of custom scripting and potential bandwidth increase from prefetching unused images is justified.
Ultimately, the choice between Astro/Image and a DIY solution depends on your project’s specific requirements and goals. It’s worth experimenting and measuring the actual impact on your website’s performance to make an informed decision.
Adding Srcset Attributes to Images
If you choose to go the DIY route for image optimization, you might also want to consider adding the srcset
attribute to your <img>
tags. This allows browsers to choose the most appropriate image based on the user’s device and screen size.
Here’s an example script to modify your HTML files and add the srcset
attribute:
const fs = require('fs');
// Function to generate srcset for different formats and sizes
function generateSrcset(src) {
const base = src.slice(0, src.lastIndexOf('.'));
const formats = ['jpg', 'webp', 'avif'];
const sizes = ['300', '600'];
let srcset = '';
formats.forEach(format => {
sizes.forEach(size => {
srcset += `${base}-${size}.${format} ${size}w, `;
});
});
// Remove the last comma and space
return srcset.slice(0, -2);
}
// Function to modify the img tag
function addSrcsetToImgTag(imgTag) {
const srcMatch = imgTag.match(/src="([^"]+)"/);
if (srcMatch && srcMatch[1]) {
const src = srcMatch[1];
const srcset = `srcset="${generateSrcset(src)}"`;
// Insert the srcset attribute into the img tag
return imgTag.replace(/<img /, `<img ${srcset} `);
}
return imgTag;
}
// Read the HTML file
fs.readFile('path/to/your/htmlfile.html', 'utf8', (err, data) => {
if (err) {
console.error('Error reading the file:', err);
return;
}
// Replace img tags using the function
const modifiedHtml = data.replace(/<img [^>]+>/g, addSrcsetToImgTag);
// Write the modified HTML back to a file
fs.writeFile('path/to/your/modified-htmlfile.html', modifiedHtml, (err) => {
if (err) {
console.error('Error writing the file:', err);
} else {
console.log('Successfully modified and saved the HTML file.');
}
});
});
This script will help you ensure that your images are displayed optimally across various devices.
Wrapping Up
In conclusion, while there are alternatives to Astro/Image for image optimization, the decision should be based on your project’s unique needs and priorities. Keep in mind that image prefetching, although useful, may not offer significant time savings for all users, and the trade-offs should be carefully considered. Whether you choose Astro/Image or opt for a custom solution, the key is to provide a fast and efficient user experience on your website.
Experiment with different approaches, measure their impact, and make an informed decision that aligns with your project’s goals. In the end, the most important thing is to create a web experience that delights your users and delivers content efficiently.
Read Count: 0