Fixing Social Share Button
Originally published: 21 August 2024
Fixing the share button shouldn't have been hard...
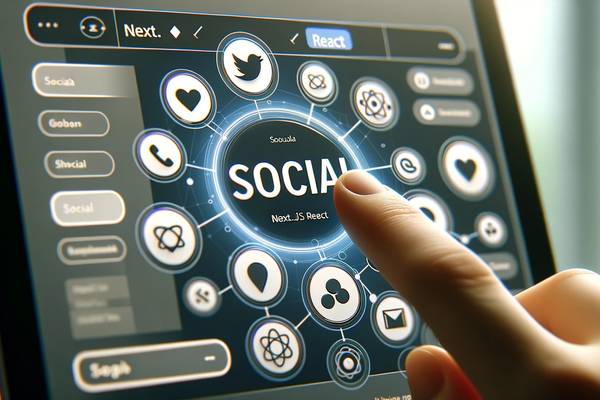
How I Fixed the Sharing Button on My Astro Website
After transitioning my website to Astro, I encountered a pesky issue with the sharing button. The button, which worked flawlessly on page load, suddenly stopped working when navigating to a new page using Astro’s smooth transitions. The problem only occurred during transitions—hard reloads or fresh page loads worked fine. Here’s how I identified and fixed the issue, and how you can do the same if you encounter a similar problem.
The Problem: Broken Sharing Button After Page Transitions
My sharing button was initially set up to function perfectly using the DOMContentLoaded
event. This event triggers when the initial HTML document has been completely loaded and parsed, which worked fine until I started using Astro’s awesome transitions.
When navigating to a new page using Astro’s built-in transitions, the button stopped working. This happened because the DOMContentLoaded
event doesn’t fire again during transitions, leaving the share button non-functional until the next hard reload.
The Fix: Leveraging the Header Logic
After much trial and error, I eventually realized that I could just use the same logic I had implemented for my header, which worked perfectly during transitions. By tapping into the astro:page-load
event, I was able to ensure that the sharing button would be reinitialized after every navigation, whether the user reloads the page or clicks through to a new one.
Here’s the final working script:
---
const share = 1;
---
<div class="flex items-center justify-center text-black mx-auto w-full overflow-visible px-5 relative">
<!-- Toggle Button for Small Screens -->
<button id={`menu-toggle-${share}`} class="p-1 shadow-xl rounded-lg border border-gray-400 hover:bg-gray-200 align-right mr-0 w-48">Share This Page</button>
<!-- Dropdown Menu -->
<div id={`dropdown-menu-${share}`} class="absolute right-0 mt-2 transform translate-x-full hidden z-20 bg-white border-gray-300 border rounded w-48 transition-transform duration-300 ease-in-out">
<a href="#" id="facebook-share" class="text-lg text-black block p-2 hover:bg-gray-100 no-underline">
<img src="/images/svg/facebook.svg" alt="Facebook" class="inline-block w-5 h-5 mr-2"> Facebook
</a>
<a href="#" id="twitter-share" class="text-lg text-black block p-2 hover:bg-gray-100 no-underline">
<img src="/images/svg/twitter.svg" alt="Twitter" class="inline-block w-5 h-5 mr-2"> Twitter
</a>
<a href="#" id="email-share" class="text-lg text-black block p-2 hover:bg-gray-100 no-underline">
<img src="/images/svg/email.svg" alt="Email" class="inline-block w-5 h-5 mr-2"> Email
</a>
</div>
</div>
<script>
const share=1;
document.addEventListener('astro:page-load', function () {
const menuToggle = document.getElementById(`menu-toggle-${share}`);
const dropdownMenu = document.getElementById(`dropdown-menu-${share}`);
const currentUrl = encodeURIComponent(window.location.href);
const currentTitle = encodeURIComponent(document.title);
const facebookShare = document.getElementById('facebook-share') as HTMLAnchorElement;
const twitterShare = document.getElementById('twitter-share') as HTMLAnchorElement;
const emailShare = document.getElementById('email-share') as HTMLAnchorElement;
// Update share links with the current URL and title
if (facebookShare) {
facebookShare.href = `https://www.facebook.com/sharer/sharer.php?u=${currentUrl}`;
}
if (twitterShare) {
twitterShare.href = `https://twitter.com/intent/tweet?url=${currentUrl}&text=${currentTitle}`;
}
if (emailShare) {
emailShare.href = `mailto:?subject=Check this page out - ${currentTitle} &body=Check out this great page I found - ${currentUrl}`;
}
// Dropdown toggle functionality
function toggleDropdown() {
if (dropdownMenu) {
dropdownMenu.classList.toggle('hidden');
}
}
if (menuToggle) {
menuToggle.addEventListener('click', (event) => {
toggleDropdown();
event.stopPropagation();
});
}
window.addEventListener('click', function (event) {
const target = event.target as Node;
if (dropdownMenu && menuToggle) {
if (!dropdownMenu.contains(target) && !menuToggle.contains(target)) {
if (!dropdownMenu.classList.contains('hidden')) {
toggleDropdown(); // Close the menu
}
}
}
});
if (dropdownMenu) {
dropdownMenu.addEventListener('click', function (event) {
event.stopPropagation();
});
}
});
</script>
Conclusion
If you’re using Astro and run into issues with components not behaving as expected during page transitions, try using the same logic you apply to your header or other persistent elements. The astro:page-load event is a reliable way to ensure that your components remain functional across transitions, saving you from the headache of elements breaking only when the user clicks through to a new page.
Read Count: 0