Add social share to your Next.js or React apps
Originally published: 31 December 2023
Use this easy social share button with Next.JS to share your pages
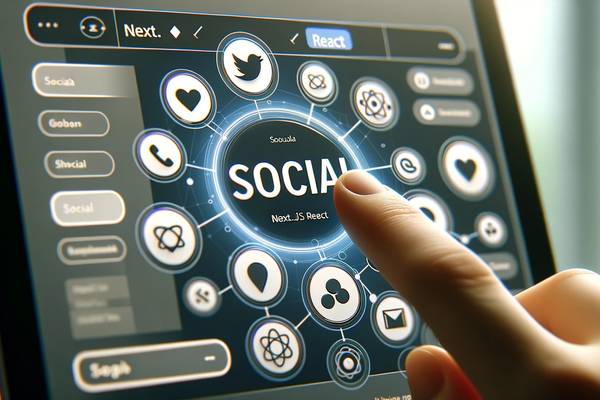
Adding Social Share Buttons to Your Next.js Blog
Allowing readers to easily share your content is crucial for driving more traffic. So let’s look at how to add customisable social sharing buttons to a Next.js blog.
Why Social Sharing Matters
Adding share buttons for platforms like Twitter, Facebook, and email enables readers to spread your content with just one click.
This makes it extremely simple for them to:
- Share posts with friends/followers
- Embed tweets to reach new visitors
- Increase your overall reach and readership
Without social sharing, readers have to manually copy links and craft posts. That extra friction means far fewer will share your work.
So making social sharing frictionless is key for capitalizing on the power of platforms like Twitter.
Creating the Share Component
To add social sharing buttons throughout our Next.js blog, we’ll create a reusable SharePage
component.
First, we’ll set up some state to track the page URL, title, and whether the share menu is open:
// share-page.js
import { useState, useEffect } from 'react';
const SharePage = () => {
const [pageUrl, setPageUrl] = useState('');
const [pageTitle, setPageTitle] = useState('');
const [showDropdown, setShowDropdown] = useState(false);
// ...
}
In useEffect
, we’ll populate the page URL and title from the browser’s window
object:
useEffect(() => {
if (typeof window !== 'undefined') {
setPageUrl(encodeURIComponent(window.location.href));
setPageTitle(encodeURIComponent(document.title));
}
}, []);
Next, we’ll define a function to toggle the dropdown open/closed, and configure share options for each social platform:
const toggleDropdown = () => setShowDropdown(!showDropdown);
const shareOptions = [
{
name: 'Twitter',
// ...
},
{
name: 'Facebook',
// ...
},
{
name: 'Email',
// ...
}
];
Then we can render the share button and conditional dropdown menu:
return (
<div>
<button onClick={toggleDropdown}>
Share This Page
</button>
{showDropdown &&
<div className="share-dropdown">
{/* Render links */}
</div>
}
</div>
);
We loop through the shareOptions
array to generate the social links, passing the page URL and title.
And that’s the core logic done! Now we can use the component and style it as needed.
Usage
To use the SharePage
component:
// SomePage.js
import SharePage from './share-page';
export default function Page() {
return (
<Layout>
<SharePage />
{/* Rest of page */}
</Layout>
)
}
Add some CSS to style the buttons, and we have a polished sharing experience ready to deploy!
Full Component Code
Here is the full SharePage
component code for reference:
// share-page.js
// Import React hooks
import { useState, useEffect } from 'react';
// SharePage component
const SharePage = () => {
// State for page URL and title
const [pageUrl, setPageUrl] = useState('');
const [pageTitle, setPageTitle] = useState('');
// Track dropdown open state
const [showDropdown, setShowDropdown] = useState(false);
// On mount, populate page URL and title
useEffect(() => {
if (typeof window !== 'undefined') {
setPageUrl(encodeURIComponent(window.location.href));
setPageTitle(encodeURIComponent(document.title));
}
}, []);
// Toggle dropdown open/closed
const toggleDropdown = () => setShowDropdown(!showDropdown);
// Share options for each platform
const shareOptions = [
{
name: 'Twitter',
url: `https://twitter.com/intent/tweet?url=${pageUrl}&text=${pageTitle}`
},
{
name: 'Facebook',
url: `https://www.facebook.com/sharer/sharer.php?u=${pageUrl}`
},
{
name: 'Email',
url: `mailto:?subject=${pageTitle}&body=${pageUrl}`
}
];
return (
<div>
{/* Share button */}
<button onClick={toggleDropdown}>
Share This Page
</button>
{/* Conditional dropdown */}
{showDropdown &&
<div className="share-dropdown">
{/* Loop through share options */}
{shareOptions.map((option, index) => (
<a
key={index}
href={option.url}
target="_blank"
rel="noopener noreferrer"
className="share-button"
>
{option.name}
</a>
))}
</div>
}
</div>
);
}
export default SharePage;
There we have it - an easy way to add social sharing buttons to any page in a Next.js app!
Addendum: Using in React Apps
While the original post focused on Next.js, this social share component code would work perfectly in any React app as well.
The core logic uses React patterns like hooks and components that work anywhere:
useState
anduseEffect
for state management- JSX for rendering UI
- Reusable component architecture
None of the code relies on Next.js-specific features.
To use the SharePage
component in a React project:
Import
and render it in your other components- Style it the same as you would any React component
- Get the page URL/title through
window.location
The only difference is the component lives where you organize React components, rather than inside pages/
like with Next.js.
But the share button functionality remains identical! This makes it a nicely reusable module for adding social sharing to any React-based application.
Read Count: 0